初めてのLaravel ④。マイグレーションのやり方
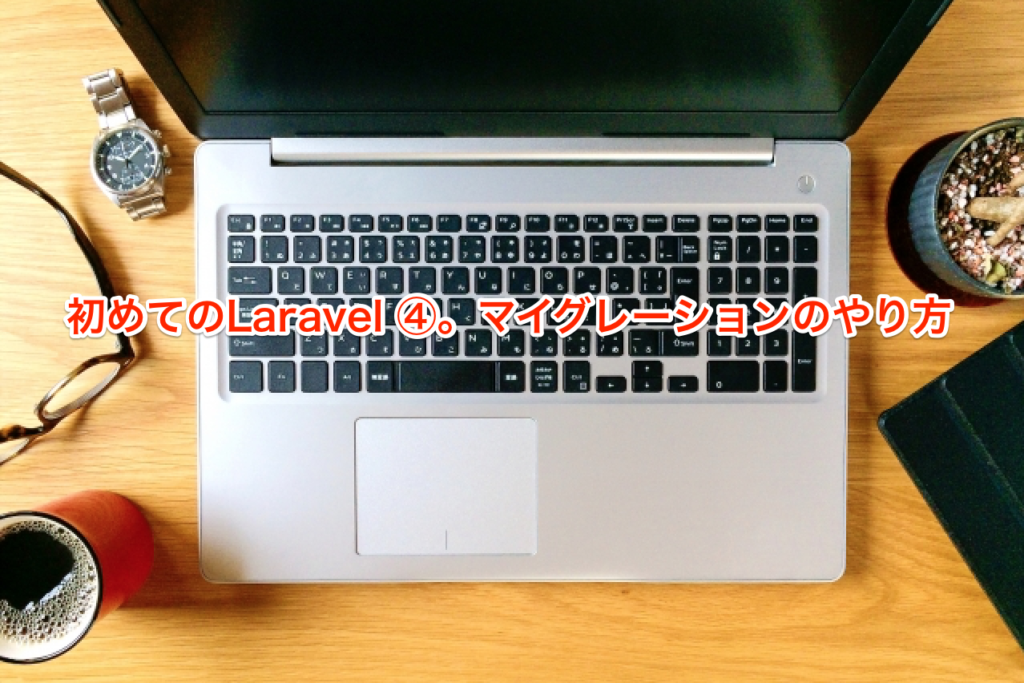
以前の記事ではTablePlusを使って手動でテーブルを作りました。Laravelではデータベースを扱う際にマイグレーションという方法も用意されています。この機能も便利ですので是非覚えておきましょう。
この記事を読む事でマイグレーションのやり方が分かります。
初めてのLaravel ④。マイグレーションのやり方
以前、TablePlusで作ったテーブルを削除してマイグレーションによりテーブルを作ってみます。
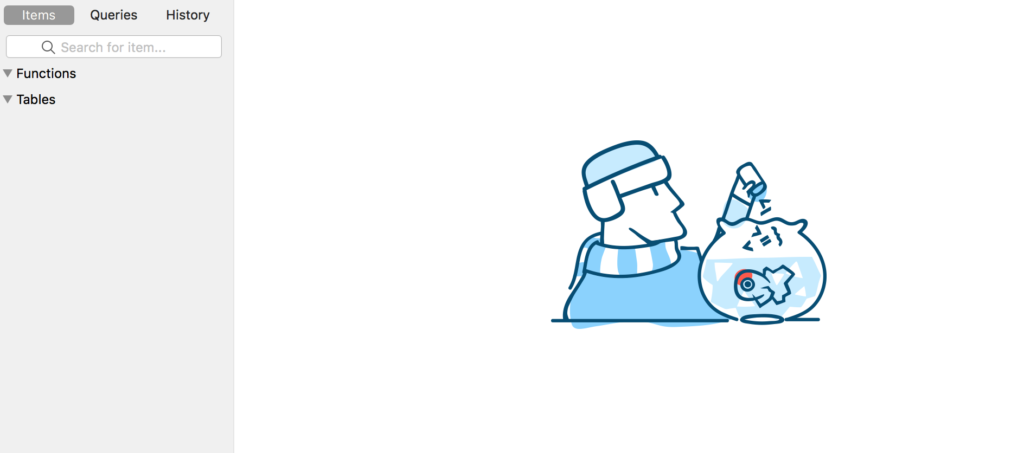
データベースに何も無い状態になりました。
続いてTerminalからcommentテーブルを作ってみます。
mbp:training user$ php artisan make:migration create_comment_table
Created Migration: 2020_06_30_121845_create_comment_table
Created Migrationと出ているのでマイグレーションファイルを確認していきます。ファイルはデータベースの中にあります。
─── database
├── migrations
├── 2020_06_30_121845_create_comment_table
作られたファイルを開くと下記のように書かれています。
class CreateCommentTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('comment', function (Blueprint $table) {
$table->id();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('comment');
}
}
up メソッドはマイグレーションを実行した時に行われるもので down はロールバックした時に行われるものです。(分かりにくいかもですが無視してもらって大丈夫です。)
では、テーブルの構成を編集していきましょう。
public function up()
{
Schema::create('comment', function (Blueprint $table) {
$table->id();
$table->text('title'); //追加
$table->string('comment'); //追加
$table->timestamps();
});
}
Terminal でマイグレーションを実行していきます。
mbp:training user$ php artisan migrate
Migration table created successfully.
Migrating: 2014_10_12_000000_create_users_table
Migrated: 2014_10_12_000000_create_users_table (0.03 seconds)
Migrating: 2014_10_12_100000_create_password_resets_table
Migrated: 2014_10_12_100000_create_password_resets_table (0.02 seconds)
Migrating: 2019_08_19_000000_create_failed_jobs_table
Migrated: 2019_08_19_000000_create_failed_jobs_table (0.01 seconds)
Migrating: 2020_06_30_121845_create_comment_table
Migrated: 2020_06_30_121845_create_comment_table (0.01 seconds)
今回作ったファイル以外にも最初から入っているファイルがあるのでそれもマイグレーションされました。
TablePlusでも確認してみます。
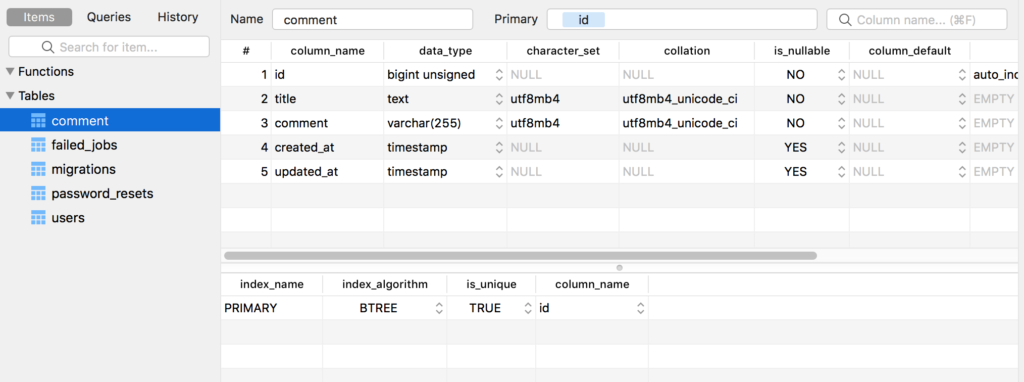
commentテーブルが作成されていますね。
テーブルの構成も予想通りになっています。
カラムの追加と削除
テーブルを作ったあとでカラムを追加したい削除したい、というときもあるでしょう。そのための方法を紹介します。
カラムの追加
まず、Terminalでカラムを追加するためのファイルを作ります。
mbp:training user$ php artisan make:migration add_body_to_comment_table
Created Migration: 2020_06_30_125453_add_body_to_comment_table
作成したマイグレーションファイルを確認します。
class AddBodyToCommentTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('comment', function (Blueprint $table) {
$table->string('body'); //追加
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('comment', function (Blueprint $table) {
$table->dropColumn('body'); //追加
});
}
commentテーブルにbodyカラムを追加、削除するコマンドを追加しました。
ちなみに、マイグレーションファイルを作った時の名前でカラムを追加するための構成にしてくれています。マイグレーションファイルを作る時には何をするためのファイルか分かりやすくしておきましょう。
では、マイグレーションを実行します。
mbp:training user$ php artisan migrate
Migrating: 2020_06_30_125453_add_body_to_comment_table
Migrated: 2020_06_30_125453_add_body_to_comment_table (0.02 seconds)
TblePlusで確認するとbodyが追加されているのが分かります。
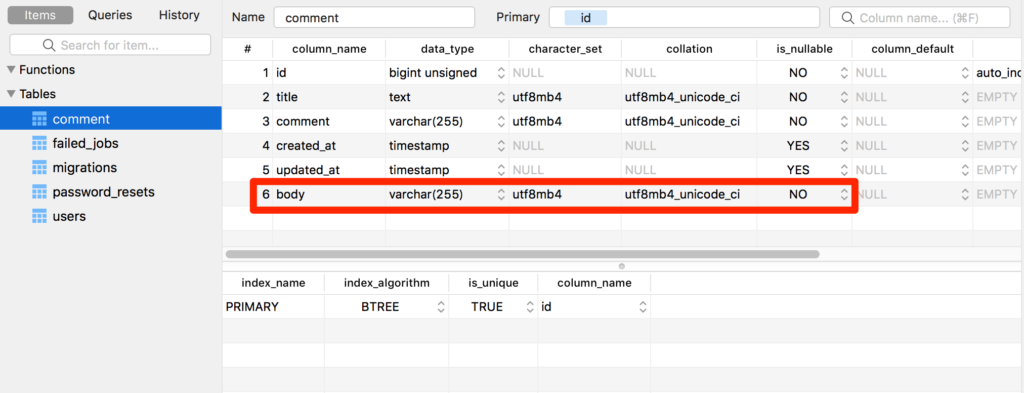
ただ、順番が最後に追加されるのでそれがいやだ、という場合は php artisan refresh で全てのマイグレーションをやり直すか、最後に->after(‘カラム名’)をつけると任意のカラムの後に追加することができます。
カラムの削除
では、rollbackでbodyカラムを削除する方法を見てみます。
すでにdownメソッドを記入したのでそのままrollbackするだけでカラムを削除できます。
mbp:training user$ php artisan migrate:rollback
Rolling back: 2020_06_30_125453_add_body_to_comment_table
Rolled back: 2020_06_30_125453_add_body_to_comment_table (0.02 seconds)
TablePlusで確認するとbodyがなくなっているのが分かります。
rollback は1つ前のマイグレーションを無効にすることを覚えておきましょう。
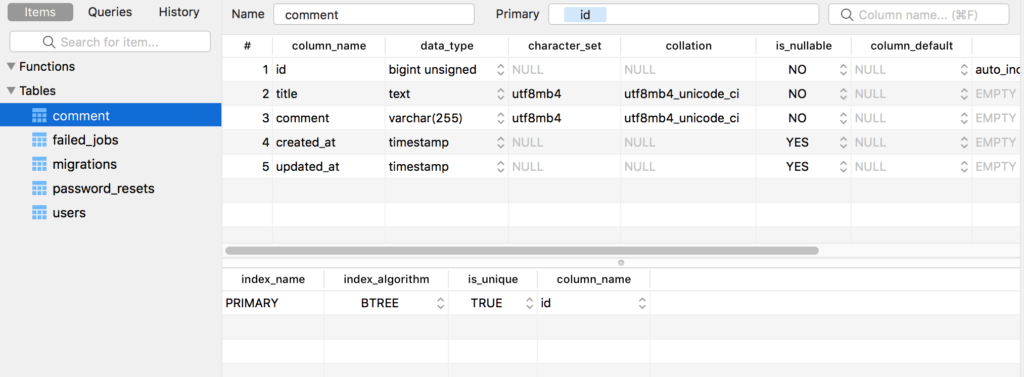
artisanコマンド
artisanコマンドを忘れてしまったというときがあるかと思います。そんな時のためにartisanコマンドはphp artisan で確認する事ができるようになっています。
mbp:training user$ php artisan
Laravel Framework 7.17.2
Usage:
command [options] [arguments]
Options:
-h, --help Display this help message
-q, --quiet Do not output any message
-V, --version Display this application version
--ansi Force ANSI output
--no-ansi Disable ANSI output
-n, --no-interaction Do not ask any interactive question
--env[=ENV] The environment the command should run under
-v|vv|vvv, --verbose Increase the verbosity of messages: 1 for normal output, 2 for more verbose output and 3 for debug
Available commands:
clear-compiled Remove the compiled class file
down Put the application into maintenance mode
env Display the current framework environment
help Displays help for a command
inspire Display an inspiring quote
list Lists commands
migrate Run the database migrations
optimize Cache the framework bootstrap files
serve Serve the application on the PHP development server
test Run the application tests
tinker Interact with your application
up Bring the application out of maintenance mode
auth
auth:clear-resets Flush expired password reset tokens
cache
cache:clear Flush the application cache
cache:forget Remove an item from the cache
cache:table Create a migration for the cache database table
config
config:cache Create a cache file for faster configuration loading
config:clear Remove the configuration cache file
db
db:seed Seed the database with records
db:wipe Drop all tables, views, and types
event
event:cache Discover and cache the application's events and listeners
event:clear Clear all cached events and listeners
event:generate Generate the missing events and listeners based on registration
event:list List the application's events and listeners
key
key:generate Set the application key
make
make:cast Create a new custom Eloquent cast class
make:channel Create a new channel class
make:command Create a new Artisan command
make:component Create a new view component class
make:controller Create a new controller class
make:event Create a new event class
make:exception Create a new custom exception class
make:factory Create a new model factory
make:job Create a new job class
make:listener Create a new event listener class
make:mail Create a new email class
make:middleware Create a new middleware class
make:migration Create a new migration file
make:model Create a new Eloquent model class
make:notification Create a new notification class
make:observer Create a new observer class
make:policy Create a new policy class
make:provider Create a new service provider class
make:request Create a new form request class
make:resource Create a new resource
make:rule Create a new validation rule
make:seeder Create a new seeder class
make:test Create a new test class
migrate //今回使ったコマンドです
migrate:fresh Drop all tables and re-run all migrations
migrate:install Create the migration repository
migrate:refresh Reset and re-run all migrations
migrate:reset Rollback all database migrations
migrate:rollback Rollback the last database migration
migrate:status Show the status of each migration
notifications
notifications:table Create a migration for the notifications table
optimize
optimize:clear Remove the cached bootstrap files
package
package:discover Rebuild the cached package manifest
queue
queue:failed List all of the failed queue jobs
queue:failed-table Create a migration for the failed queue jobs database table
queue:flush Flush all of the failed queue jobs
queue:forget Delete a failed queue job
queue:listen Listen to a given queue
queue:restart Restart queue worker daemons after their current job
queue:retry Retry a failed queue job
queue:table Create a migration for the queue jobs database table
queue:work Start processing jobs on the queue as a daemon
route
route:cache Create a route cache file for faster route registration
route:clear Remove the route cache file
route:list List all registered routes
schedule
schedule:run Run the scheduled commands
session
session:table Create a migration for the session database table
storage
storage:link Create the symbolic links configured for the application
stub
stub:publish Publish all stubs that are available for customization
vendor
vendor:publish Publish any publishable assets from vendor packages
view
view:cache Compile all of the application's Blade templates
view:clear Clear all compiled view files
コマンドを忘れてしまった、という時にはphp artisanで確認するようにしましょう。いろんなコマンドがあるので暇な問いに眺めておくと良いでしょう。
まとめ
今回はマイグレーションの基本的なやり方を紹介しました。
TablePlusでMySQLが簡単に扱えますがそれよりもさらに簡単に扱えますね。最初は不便に感じるかもしれませんが慣れればマイグレーションの方が早くなります。